Making a reporter
Anything that only works unsandboxed will be noted, but regardless, we will assume you are creating an unsandboxed extension during this tutorial.
Make a new block
Add a new object to the blocks
array. This time, the blockType
should be Scratch.BlockType.REPORTER
.
Let's say this block will just use a simple testReporter()
function:
[
{
opcode: 'logToConsole',
text: 'log to console',
blockType: Scratch.BlockType.COMMAND
},
{
opcode: 'testReporter',
text: 'testing!',
blockType: Scratch.BlockType.REPORTER
}
]
And let's just make the function return a basic string:
class Extension {
// getInfo...
logToConsole() {
console.log('Hello world!');
}
testReporter() {
return "Hello world!";
}
}
Loading this into Snail IDE, you should see the following:
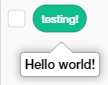
This works! But why is there a checkbox?
Reporter-specific parameters
disableMonitor
We can add the disableMonitor
property to our block to remove the variable monitor option in the category.

{
opcode: 'testReporter',
text: 'testing!',
blockType: Scratch.BlockType.REPORTER,
disableMonitor: true
}
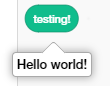
This isn't the only specific parameter we can add here:
allowDropAnywhere
This is used a lot less, but we can use this parameter to place the block into "unexpected areas":
{
opcode: 'testReporter',
text: 'testing!',
blockType: Scratch.BlockType.REPORTER,
allowDropAnywhere: true
}
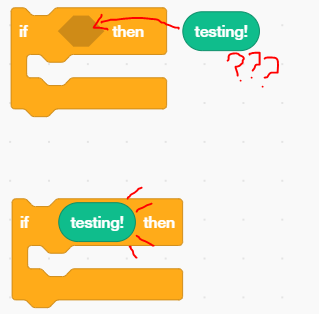
Final code
first-reporter.js - View source
(function(Scratch) {
'use strict';
class Extension {
getInfo() {
return {
id: "johnMyExtension",
name: "My Extension",
blocks: [
{
opcode: 'logToConsole',
text: 'log to console',
blockType: Scratch.BlockType.COMMAND
},
{
opcode: 'testReporter',
text: 'testing!',
blockType: Scratch.BlockType.REPORTER,
disableMonitor: true,
allowDropAnywhere: true
}
]
};
}
logToConsole() {
console.log('Hello world!');
}
testReporter() {
return "Hello world!";
}
}
Scratch.extensions.register(new Extension());
})(Scratch);
Next steps
Why don't we create a real boolean next?